Cards
Bootstrap’s cards provide a flexible and extensible content container with multiple variants and options.
Custom Cards
Pre-built cards for copygen.
Social Media Post
Hey everyone! Have you met ChatGPT? As an AI language model, I'm trained to answer your questions and have a conversation with you! Ask me anything, and let's get chatting! 😊
Hey everyone! Have you met ChatGPT? As an AI language model, I'm trained to answer your questions and have a conversation with you! Ask me anything, and let's get chatting! 😊
Blog Ideas
Produce trendy blog ideas for your business that engages.
import CustomCardStyle2 from '@/app/ui/CustomCard/CustomCardStyle2';
import CustomCardStyle3 from '@/app/ui/CustomCard/CustomCardStyle3';
import CustomCardStyle4 from '@/app/ui/CustomCard/CustomCardStyle4';
import CustomCardStyle5 from '@/app/ui/CustomCard/CustomCardStyle5';
export default function Example() {
return (
<Row className="g-gs">
<Col lg="6">
<CustomCardStyle2
category="Social Media Post"
icon="pen-fill"
iconBg="bg-primary text-primary"
text="Hey everyone! Have you met ChatGPT? As an AI language model, I'm trained to answer your questions and have a conversation with you! Ask me anything, and let's get chatting! 😊"
time="5:22 PM"
wordNumber="29"
characterNumber="129"
/>
</Col>
<Col lg="6">
<CustomCardStyle3
category="Social Media Post"
text="Hey everyone! Have you met ChatGPT? As an AI language model, I'm trained to answer your questions and have a conversation with you! Ask me anything, and let's get chatting! 😊"
date="Feb 23, 2023"
time="05:23 PM"
wordNumber={42}
/>
</Col>
<Col lg="3">
<CustomCardStyle4
title="Blog Ideas"
subTitle="Produce trendy blog ideas for your business that engages."
icon="bulb-fill"
iconBgClass="text-primary bg-primary"
newBadge
/>
</Col>
<Col lg="4">
<CustomCardStyle5
title="Words Available"
btnText="See History"
btnHref="/history"
numbers={452}
numbersText="words"
percentage={74}
cardFooerNumber={1548}
cardFooerText="/2000 free words generated"
/>
</Col>
</Row>
);
}
Bootstrap Cards
Follow the codebase for bootstrap basic card
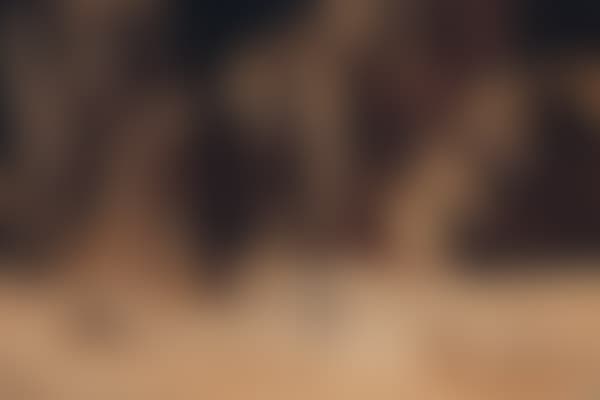
Some quick example text to build on the card title and make up the bulk of the card's content.
import {Button, Card} from 'react-bootstrap';
import Image from 'next/image';
export default function BasicExample() {
return (
<Card style={{ width: '18rem' }}>
<Image src="/images/preview/b.jpg" width={600} height={400} className="card-img-top h-auto" />
<Card.Body>
<Card.Title>Card Title</Card.Title>
<Card.Text>
Some quick example text to build on the card title and make up the
bulk of the card's content.
</Card.Text>
<Button variant="primary">Go somewhere</Button>
</Card.Body>
</Card>
);
}
Body
Use <Card.Body> to pad content inside a <Card>.
import {Card} from 'react-bootstrap';
export default function BodyOnlyExample() {
return (
<Card>
<Card.Body>This is some text within a card body.</Card.Body>
</Card>
);
}
Title, text, and links
Using <Card.Title>, <Card.Subtitle>, and <Card.Text> inside the <Card.Body> will line them up nicely. <Card.Link>s are used to line up links next to each other. <Card.Text> outputs <p> tags around the content, so you can use multiple <Card.Text>s to create separate paragraphs.
Some quick example text to build on the card title and make up the bulk of the card's content.
Card LinkAnother Linkimport {Card} from 'react-bootstrap';
export default function TextExample() {
return (
<Card>
<Card.Body>
<Card.Title>Card Title</Card.Title>
<Card.Subtitle className="mb-2 text-muted">Card Subtitle</Card.Subtitle>
<Card.Text>
Some quick example text to build on the card title and make up the
bulk of the card's content.
</Card.Text>
<Card.Link href="/">Card Link</Card.Link>
<Card.Link href="/">Another Link</Card.Link>
</Card.Body>
</Card>
);
}
Kitchen sink
Follow the codebase for bootstrap kitchen sink
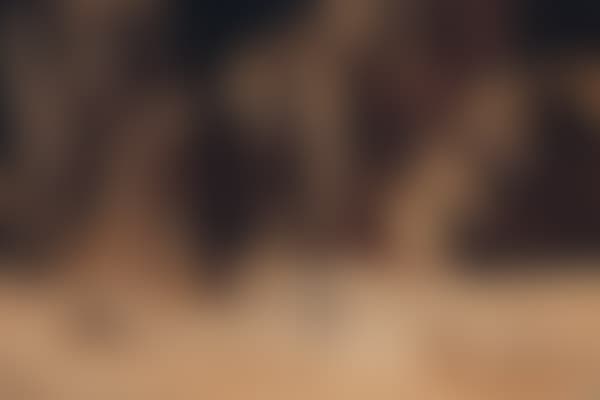
Some quick example text to build on the card title and make up the bulk of the card's content.
import { Card, Col, ListGroup, Row } from 'react-bootstrap';
import Image from 'next/image';
export default function KitchenSinkExample() {
return (
<Row className="g-gs">
<Col md="6" lg="4">
<Card>
<Image
src="/images/preview/b.jpg"
width={600}
height={400}
className="card-img-top h-auto"
/>
<Card.Body>
<Card.Title>Card Title</Card.Title>
<Card.Text>
Some quick example text to build on the card title and make up
the bulk of the card's content.
</Card.Text>
</Card.Body>
<ListGroup className="list-group-flush">
<ListGroup.Item>Cras justo odio</ListGroup.Item>
<ListGroup.Item>Dapibus ac facilisis in</ListGroup.Item>
<ListGroup.Item>Vestibulum at eros</ListGroup.Item>
</ListGroup>
<Card.Body>
<Card.Link href="#">Card Link</Card.Link>
<Card.Link href="#">Another Link</Card.Link>
</Card.Body>
</Card>
</Col>
</Row>
);
}
Header and footer
Add an optional header and/or footer within a card.
With supporting text below as a natural lead-in to additional content.
import { Button, Card, Col, Row } from 'react-bootstrap';
export default function HeaderAndFooterExample() {
return (
<Row className="g-gs">
<Col lg="6">
<Card>
<Card.Header>Featured</Card.Header>
<Card.Body>
<Card.Title>Special title treatment</Card.Title>
<Card.Text>
With supporting text below as a natural lead-in to additional
content.
</Card.Text>
<Button variant="primary">Go somewhere</Button>
</Card.Body>
<Card.Footer className="text-muted">2 days ago</Card.Footer>
</Card>
</Col>
</Row>
);
}
Header and footer
Add an optional header and/or footer within a card.
Hey everyone! Have you met ChatGPT? As an AI language model, I'm trained to answer your questions and have a conversation with you! Ask me anything, and let's get chatting! 😊
Hey everyone! Have you met ChatGPT? As an AI language model, I'm trained to answer your questions and have a conversation with you! Ask me anything, and let's get chatting! 😊
import { Button, Card, Col, Row } from 'react-bootstrap';
export default function UsingUtilitiesExample() {
return (
<>
<Row className="mb-4">
<Col lg="9">
<Card>
<Card.Body>
<Card.Title>Card title</Card.Title>
<Card.Text>
Hey everyone! Have you met ChatGPT? As an AI language model,
I'm trained to answer your questions and have a conversation
with you! Ask me anything, and let's get chatting! 😊
</Card.Text>
<Button variant="primary">Button</Button>
</Card.Body>
</Card>
</Col>
</Row>
<Row>
<Col lg="6">
<Card>
<Card.Body>
<Card.Title>Card title</Card.Title>
<Card.Text>
Hey everyone! Have you met ChatGPT? As an AI language model,
I'm trained to answer your questions and have a conversation
with you! Ask me anything, and let's get chatting! 😊
</Card.Text>
<Button variant="primary">Button</Button>
</Card.Body>
</Card>
</Col>
</Row>
</>
);
}
Text alignment
Add an optional header and/or footer within a card.
With supporting text below as a natural lead-in to additional content.
With supporting text below as a natural lead-in to additional content.
With supporting text below as a natural lead-in to additional content.
import { Button, Card, Col, Row } from 'react-bootstrap';
export default function UsingUtilitiesExample() {
return (
<>
<Row className="mb-4">
<Col lg="3">
<Card>
<Card.Body>
<Card.Title>Special title treatment</Card.Title>
<Card.Text>
With supporting text below as a natural lead-in to additional
content.
</Card.Text>
<Button variant="primary">Go somewhere</Button>
</Card.Body>
</Card>
</Col>
<Col lg="3">
<Card className="text-center">
<Card.Body>
<Card.Title>Special title treatment</Card.Title>
<Card.Text>
With supporting text below as a natural lead-in to additional
content.
</Card.Text>
<Button variant="primary">Go somewhere</Button>
</Card.Body>
</Card>
</Col>
<Col lg="3">
<Card className="text-end">
<Card.Body>
<Card.Title>Special title treatment</Card.Title>
<Card.Text>
With supporting text below as a natural lead-in to additional
content.
</Card.Text>
<Button variant="primary">Go somewhere</Button>
</Card.Body>
</Card>
</Col>
</Row>
</>
);
}
Navigation
Add some navigation to a card’s header (or block) with React Bootstrap’s Nav components.
With supporting text below as a natural lead-in to additional content.
import {Button, Card, Nav} from 'react-bootstrap';
export default function NavTabsExample() {
return (
<Card>
<Card.Header>
<Nav variant="tabs" defaultActiveKey="#first">
<Nav.Item>
<Nav.Link href="#first">Active</Nav.Link>
</Nav.Item>
<Nav.Item>
<Nav.Link href="#link">Link</Nav.Link>
</Nav.Item>
<Nav.Item>
<Nav.Link href="#disabled" disabled>
Disabled
</Nav.Link>
</Nav.Item>
</Nav>
</Card.Header>
<Card.Body className="text-center">
<Card.Title>Special title treatment</Card.Title>
<Card.Text>
With supporting text below as a natural lead-in to additional content.
</Card.Text>
<Button variant="primary">Go somewhere</Button>
</Card.Body>
</Card>
);
}
Image caps
Similar to headers and footers, cards can include top and bottom “image caps”—images at the top or bottom of a card.
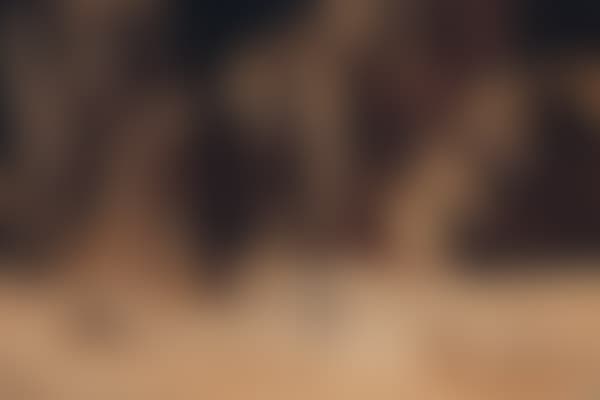
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
Last updated 3 mins ago
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
Last updated 3 mins ago
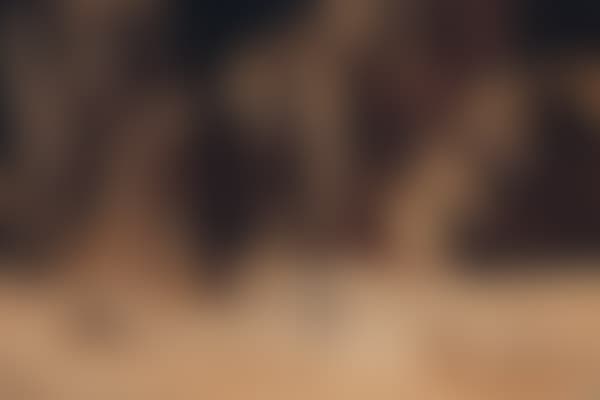
import {Button, Card, Nav} from 'react-bootstrap';
import Image from 'next/image';
export default function NavTabsExample() {
return (
<Row>
<Col lg="3">
<Card>
<Image src="/images/preview/b.jpg" width={600} height={400} className="card-img-top h-auto" />
<Card.Body>
<Card.Title>Card title</Card.Title>
<Card.Text>
This is a wider card with supporting text below as a natural
lead-in to additional content. This content is a little bit longer.
</Card.Text>
<Card.Text>
<small className="text-muted">Last updated 3 mins ago</small>
</Card.Text>
</Card.Body>
</Card>
</Col>
<Col lg="3">
<Card>
<Card.Body>
<Card.Title>Card title</Card.Title>
<Card.Text>
This is a wider card with supporting text below as a natural
lead-in to additional content. This content is a little bit longer.
</Card.Text>
<Card.Text>
<small className="text-muted">Last updated 3 mins ago</small>
</Card.Text>
</Card.Body>
<Image src="/images/preview/b.jpg" width={600} height={400} className="card-img-top h-auto" />
</Card>
</Col>
</Row>
);
}
Image overlays
Turn an image into a card background and overlay your card’s text. Depending on the image, you may or may not need additional styles or utilities.
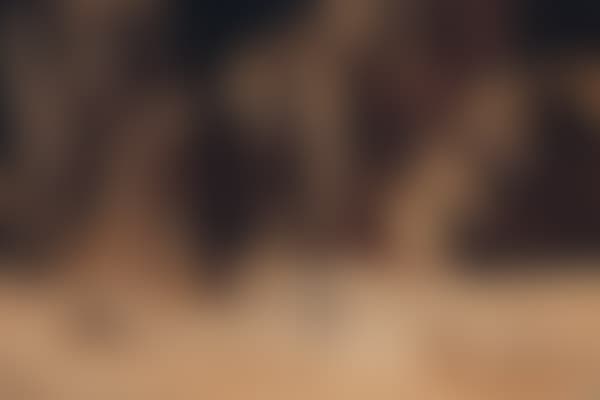
import {Row, Col, Card} from 'react-bootstrap';
import Image from 'next/image';
export default function Example() {
return (
<Row>
<Col lg="4">
<Card className="bg-dark text-white">
<Image src="/images/preview/b.jpg" width={600} height={400} className="card-img h-auto" />
<Card.ImgOverlay>
<Card.Title>Card title</Card.Title>
<Card.Text>
This is a wider card with supporting text below as a natural
lead-in to additional content. This content is a little bit
longer.
</Card.Text>
<Card.Text>Last updated 3 mins ago</Card.Text>
</Card.ImgOverlay>
</Card>
</Col>
</Row>
);
}
Horizontal
Follow the codebase for bootstrap basic card
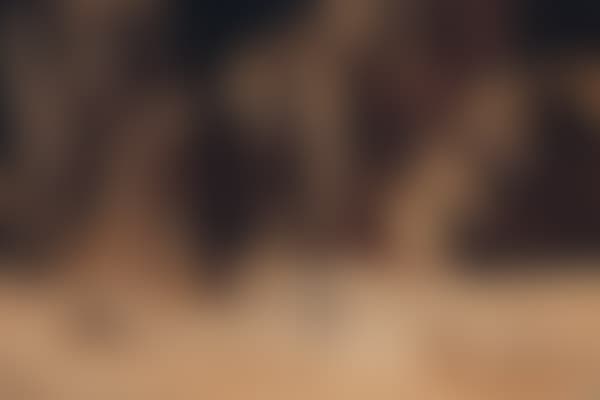
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
Last updated 3 mins ago
import {Button, Card, Row, Col} from 'react-bootstrap';
import Image from 'next/image';
export default function BasicExample() {
return (
<Card>
<Row className="g-0">
<Col md="4">
<Image src="/images/preview/b.jpg" width={600} height={400} className="rounded-start h-100" />
</Col>
<Col md="8">
<Card.Body>
<Card.Title>Card title</Card.Title>
<Card.Text>
This is a wider card with supporting text below as a natural
lead-in to additional content. This content is a little bit
longer.
</Card.Text>
<Card.Text>
<small className="text-muted">Last updated 3 mins ago</small>
</Card.Text>
</Card.Body>
</Col>
</Row>
</Card>
);
}
Grid cards
Use Row's grid column props to control how many cards to show per row.
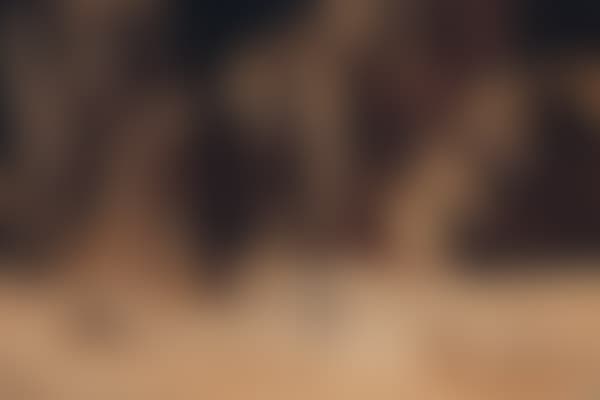
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
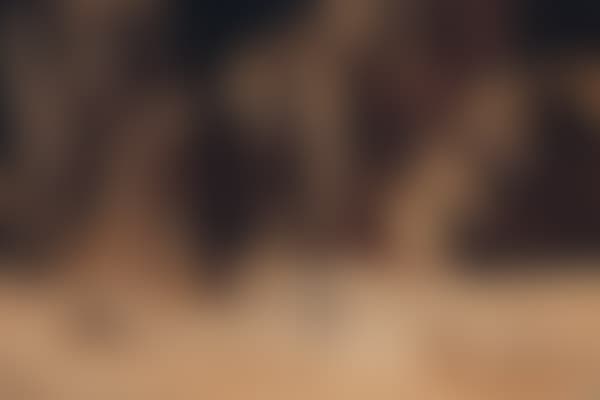
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
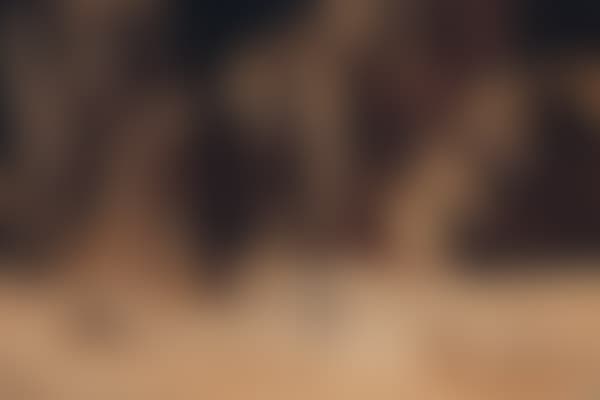
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
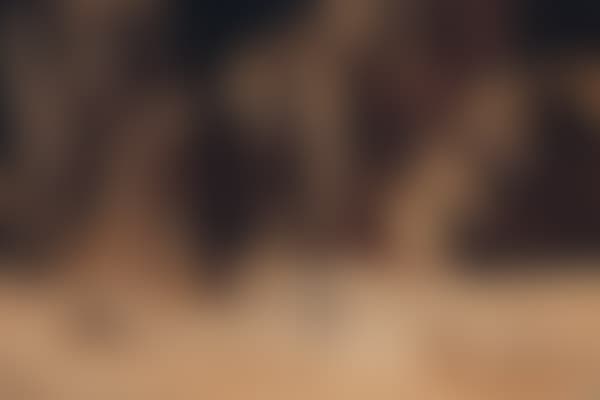
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
import {Card, Row, Col} from 'react-bootstrap';
import Image from 'next/image';
export default function BasicExample() {
return (
<Row xs={1} md={2} className="g-4">
{Array.from({ length: 4 }).map((_, idx) => (
<Col key={idx}>
<Card>
<Image
src="/images/preview/b.jpg"
width={600}
height={400}
className="card-img-top h-100"
/>
<Card.Body>
<Card.Title>Card title</Card.Title>
<Card.Text>
This is a longer card with supporting text below as a
natural lead-in to additional content. This content is a
little bit longer.
</Card.Text>
</Card.Body>
</Card>
</Col>
))}
</Row>
);
}